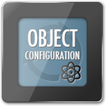
Scripting with Dates¶
Initial Situation
- 2 read-only attributes with different data types: Results[Text] and Results_Date[Date]
- 1 attribute Calculations [Text] for calculating both read-only attributes
toDate() or toJavaDate()?¶
Sample Code in the Calculations field
var d1 = new Date();
//#1
obj.set('Results',d1);
// #2
obj.set('Results',toStr(d1);
// #3
obj.set('Results_Date',toDate(d1));
//#4
obj.set('Results_Date',toJavaDate(d1));
Code Comment
- #1:
- new Date() will generate a new JavaScript Date.
- Writing it to a [Text] field produces an error
- Error: “NativeDate cannot be cast to java.lang.String…”
- #2:
- Type cast d1 to [Text]. Feeder will allow saving in the Results[Text] field
- #3:
- Type cast d1 to a JavaScript [Date]. Feeder will deny saving in the Results_Date[Date] field!
- Error: “NativeDate can’t be recognized as date”
- #4:
- Type cast your JavaScript Date to a Java Date. Feeder will allow saving in Results_Date[Date]
Working with Date Attributes (obj.get(…))¶
Sample Code
var d2 = obj.get('DATE_OF_BIRTH');
// #5a
obj.set('Results', d2);
// #5b
obj.set('Results', toStr(d2);
// #6a
obj.set('Results_Date', d2);
// #6b
obj.set('Results_Date', toDate(d2);
Code Comment Date Retrieval
- #5a:
- Produces an error (LocalDate cannot be cast to java.lang.String)
- #5b:
- Prints 1986-11-09
- #6a:
- Will print and display the date in the Date pattern associated with the Feeder user language setting (e.g. English -> 11/05/1986, German -> 09.11.1986).
- obj.set(‘Results_Date’, toJavaDate(d2) would have the same effect
- #6b:
- Produces an error (the current value [..], can’t be recognized as date)
Comparing JavaScript Dates¶
Sample Code
var dob = obj.get('DATE_OF_BIRTH');
var today = new Date();
//#a
obj.set('Results', 'dob: '+ dob + ' today: ' + today);
//#b
obj.set('Results', 'dob: '+ toDate(dob) + ' today: ' + today);
//#c
obj.set('Results', '<: '+ (toDate(dob).getTime() < today.getTime()));
//#d
obj.set('Results', '<: '+ (toDate(dob).getTime() == today.getTime()));
//#e
today.setDate(today.getDate() - 7);
Code Comment Comparing JavaDates
- #a:
- dob: 1986-07-09
- today: Thu Nov 05 2015 16:54:07 GMT+0100 (CET)
- #b:
- dob: Wed Jul 09 1986 00:00:00 GMT+0200 (CEST)
- today: Thu Nov 05 2015 16:53:21 GMT+0100 (CET)
- #c:
- Will be”true”. Beware the value is a Text/ String!
- #d:
- Will be”false”. Beware the value is a Text/ String!
- #e:
- Substracts 7 days from today